ThemeAPI - Api for third-party JavaScript running in the chat browser application on the
visitor's page.
Below an example of custom JavaScript as part of a yalst theme file is shown.
Below an example of custom JavaScript as part of a yalst theme file is shown.
- Version:
- 1.1
- Copyright:
- Visisoft OHG 2015
- Source:
Example
function themeApiReady()
{
var startTime, quitTime, opName, chatId;
require(['themeApi'], function(themeApi)
{
// subscribe for all chat events
var subscription = themeApi.chatEventPublisher.subscribe(
'chat',
function(event, data)
{
if (event == "chat.pending"){
startTime = Date.now();
chatId = data.chatId;
}
else if (event == "chat.joined"){
opName = data.operatorName;
}
}
);
// subscribe in particular for "chat closed" event
themeApi.chatEventPublisher.subscribe(
'chat.closed',
function(event, data)
{
if (data.reason == "visitor"){
console.log("The visitor quit.");
}
else if (data.reason == "operator"){
console.log("The operator quit.");
}
/// clean up
subscription.dispose();
}
);
});
}
Members
(static) chatEventPublisher :module:lib/pubsub~Publisher
Publisher will emit key events during a chat procedure
Type:
- Source:
Fires:
- module:themeApi.event:"chat"
- module:themeApi.event:"chat.pending"
- module:themeApi.event:"chat.joined"
- module:themeApi.event:"chat.closed"
- module:themeApi.event:"chat.closing"
- module:themeApi.event:"chat.refused"
- module:themeApi.event:"chat.paused"
- module:themeApi.event:"chat.resumed"
- module:themeApi.event:"chat.re-rendered"
- module:themeApi.event:"pageChange"
- module:themeApi.event:"sendMessage"
- module:themeApi.event:"receivedMessage"
- module:themeApi.event:"startFormValidated"
(static, readonly) PageId :string
Enumeration of the application pages (views) presented to the user.
Used by the module:themeApi.event:"pageChange" event.
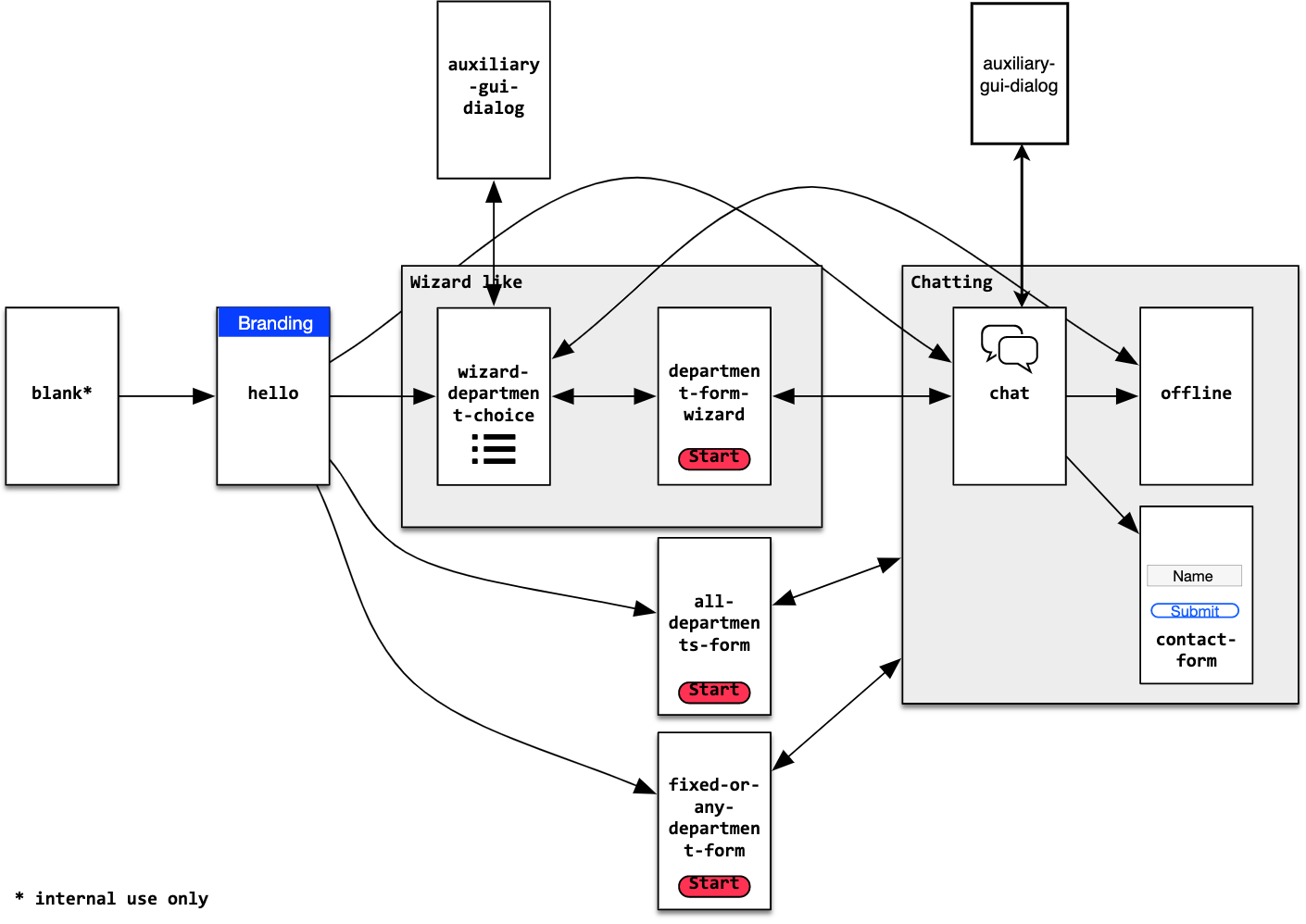
Type:
- string
Properties:
Name | Type | Description |
---|---|---|
blank |
string | Internal use only "blank" - initial page shown while the loading resource files from the network or browser cache |
hello |
string | "hello" - intermediate page with (optional) logo images and welcome phrases, shown before the welcoming forms are loaded |
"wizard-department-choice" |
string | "wizard-department-choice" - choice of departments is shown when the welcome forms differ between departments |
"department-form-wizard" |
string | "department-form-wizard" - welcoming form of a particular department |
chat |
string | "chat" - the chat view |
offline |
string | "offline" - a predefined offline text |
"contact-form" |
string | "contact-form" - a page with the contact form can be displayed if the chat is offline or the operator could not pick up the chat request in time |
"all-departments-form" |
string | "all-departments-form" - the common welcome form for all departments with a department selector |
"fixed-or-any-department-form" |
string | "fixed-or-any-department-form" - a welcoming form for a particular department or any department |
"faq-root" |
string | "faq-root" - Root page of the FAQ pages |
"auxiliary-gui-dialog" |
string | "auxiliary-gui-dialog" - an internal gui helper page e.g. when a long custom (non-native) select box is displayed on an extra page by jQuery Mobile |
- Source:
Example
require(['themeApi'], function(themeApi)
{
themeApi.chatEventPublisher.subscribe(
"pageChange",
function(event, info)
{
// "blank"->"hello"->"wizard-department-choice"
console.log("page:" + info.pageId);
// e.g. "B" or undefined
console.log("department:" + info.departmentId);
});
});
(static, readonly) QuitReason :string
Enumeration of reasons for chat termination.
Given by the module:themeApi.event:"chat.closed" event.
Type:
- string
Properties:
Name | Type | Description |
---|---|---|
visitor |
string | "visitor" - the visitor has terminated the chat |
operator |
string | "operator" - the operator has terminated the chat |
system |
string | "system" - unknown reason |
timeout |
string | 'timeout' - !Not implemented! the chat was terminated by the system because there was no activity for a longer period in time |
- Source:
(static, readonly) RefuseReason :string
Enumeration of reasons for chat termination.
Given by the module:themeApi.event:"chat.refused" event.
Type:
- string
Properties:
Name | Type | Description |
---|---|---|
refused |
string | "refused" - an operator did decline the chat request in the operator console |
blocked |
string | "blocked" - an operator did block the user in the operator console |
timeout |
string | 'timeout' - The chat was not joined by any operator withing the maximum wait time |
- Source:
(static) version :string
api version number e.g. "1.0.0+1808"
Type:
- string
- Source:
Events
event:"chat.closed"
Event fires when the chat gets finished.
Type:
- object
Properties:
Name | Type | Description |
---|---|---|
reason |
module:themeApi.QuitReason | The reason for quitting the chat. |
- Source:
event:"chat.closing"
Event fires when the visitor has decided to close the chat.
When the close command is received by the chat server this event
will be followed by the module:themeApi.event:"chat.closed" event
- Since:
- 1.1
- Source:
event:"chat.joined"
Event fires when an operator/agent has picked up the chat request from the user.
Type:
- object
Properties:
Name | Type | Attributes | Description |
---|---|---|---|
operatorName |
string | The name of the associated operator. | |
infoLine |
string |
<optional> |
String with more details about the associated operator. |
chatId |
string | The ID of the new chat. | |
sessionId |
string | The session ID of the new chat |
- Source:
event:"chat.paused"
Event fires when a chat is pending or running, right before the web app will be hibernated by the browser.
Important: You must execute
only synchronous (blocking) JavaScript instructions in your handler for
this event!
- Source:
event:"chat.pending"
Event fires when the user initiates a chat by tapping on the start button
if the selected live support department or service is not busy or offline.
Type:
- object
Properties:
Name | Type | Description |
---|---|---|
chatId |
string | The ID of the new chat. |
sessionId |
string | The session ID of the new chat |
- Source:
event:"chat.re-rendered"
Event fires when the browser re-renders a closed chat
Type:
- object
Properties:
Name | Type | Description |
---|---|---|
chatId |
string | The ID of the new chat. |
sessionId |
string | The session ID of the new chat |
- Source:
event:"chat.refused"
Event fires when no operator will join the pending chat.
Type:
- object
Properties:
Name | Type | Description |
---|---|---|
reason |
module:themeApi.RefuseReason | The reason for refusing the chat. |
- Source:
event:"chat.resumed"
Event fires when the browser revives the formally hibernated web app being pending or running
Properties:
Name | Type | Attributes | Description |
---|---|---|---|
operatorName |
string | The name of the associated operator. | |
infoLine |
string |
<optional> |
String with more details about the associated operator. |
- Source:
event:"chat"
High level event. Encompasses all special chat events.
Type:
- object
- Source:
event:"pageChange"
Event fires when a new page (view) is shown
Type:
- object
Properties:
Name | Type | Description |
---|---|---|
pageId |
module:themeApi.PageId | the new page identifier |
departmentId |
string | undefined | The department selected by the visitor. |
- Source:
event:"receivedMessage"
Event fires when a chat contribution is received from the operator or the chat system.
Type:
- object
Properties:
Name | Type | Description |
---|---|---|
text |
string | undefined | The transmitted text. Undefined if a file or image |
origin |
string | undefined | The originator , "operator", "system" or "visitor" |
- Since:
- 1.1
- Source:
event:"sendMessage"
Event fires when the user hits the Send Button in a chat.
Type:
- object
Properties:
Name | Type | Description |
---|---|---|
text |
string | undefined | The text typed in bx the user. Undefined if a file or image was posted instead. |
- Source:
event:"startFormValidated"
Event fires when the start/welcome form is validated with success on the user hitting the start chat button.
Type:
- object
Properties:
Name | Type | Description |
---|---|---|
departmentId |
string | undefined | The department which provides the start form. |
- Source: